Sprites and Distances
/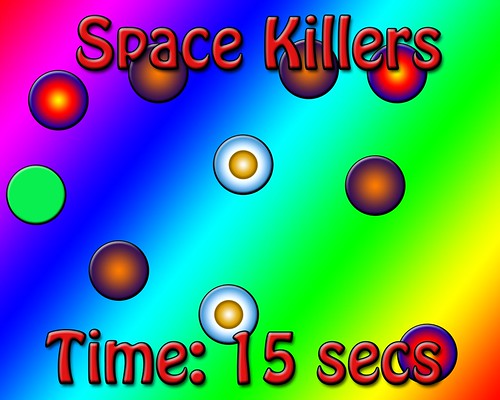
At the moment quite a few of the first year are working on a “Space Killers” game. Above you can see my sample screenshot from the specification. You can see that my graphical design abilities are around the same as usual.
The idea is that the player (the green object) must survive in brightly coloured space while all around are trying to kill. Collision with any game object is instant death. Survive until the clock counts down to zero and you are rewarded with another level with more, and nastier, things in it.
One of the game objects is the “Master”. This is the one that looks a bit like a fried egg in the above screenshot. (Note to graphics department, fix that please).
Anyhoo, a Master sprite will sit and do nothing unless you get too close. Then it wakes up and chases you. To make this work the game needs to be able to work out how far the master is from the player. In my solution I have a sprite class:
public class Sprite { public Texture2D SpriteTexture; public Rectangle SpriteRectangle; public Vector2 SpritePosition; public Color SpriteColor; }
This contains stuff that we need to position and draw the sprite. The Update method for the parent sprite just uses the position vector (which I use to decide where the sprite is on the screen) to set the SpriteRectangle value (which I use to decide where to draw the sprite).
public virtual void Update(SpaceKillersGame game) { SpriteRectangle.X = (int)(SpritePosition.X + 0.5f); SpriteRectangle.Y = (int)(SpritePosition.Y + 0.5f); }
My sprite also contains another method, DistanceFrom, which is used by code in the Master sprite to decide how close it is to the player.
public float DistanceFrom(Sprite otherSprite) { float dx = SpriteRectangle.Center.X - otherSprite.SpriteRectangle.Center.X; float dy = SpriteRectangle.Center.Y - otherSprite.SpriteRectangle.Center.Y; return (float) Math.Sqrt((dx * dx) + (dy * dy)); }
This is how it works. It turns out that the Rectangle class contains a Point value which gives the coordinates of the centre of the rectangle. I can use the X and Y components from this to work out the difference in x and y between the two rectangles. I can then use Pythagoras Theorem to work out the distance between the sprites. Pythagoras said “The sum of the squares of the sides of a right angled triangle is equal to the square of the hypotenuse”. My code works out the sum of the squares and then takes the square root of this to work out the distance value.
Hopefully the above diagram will make it all clear. If not, just enjoy the pretty colours.
My Master sprite then contains the following statement in its Update method (Update is called on the sprite to make it update itself. It is given a reference to the game that is currently active).
if (DistanceFrom(game.Player) < MasterRadarRange) { ChaseActive = true; }MasterRadarRange is a value which controls how far the Master can “see”. If a player gets closer than the radar range the flag ChaseActive is set to true, which triggers the chase behaviour in the Master sprite.